Marginally better stack traces
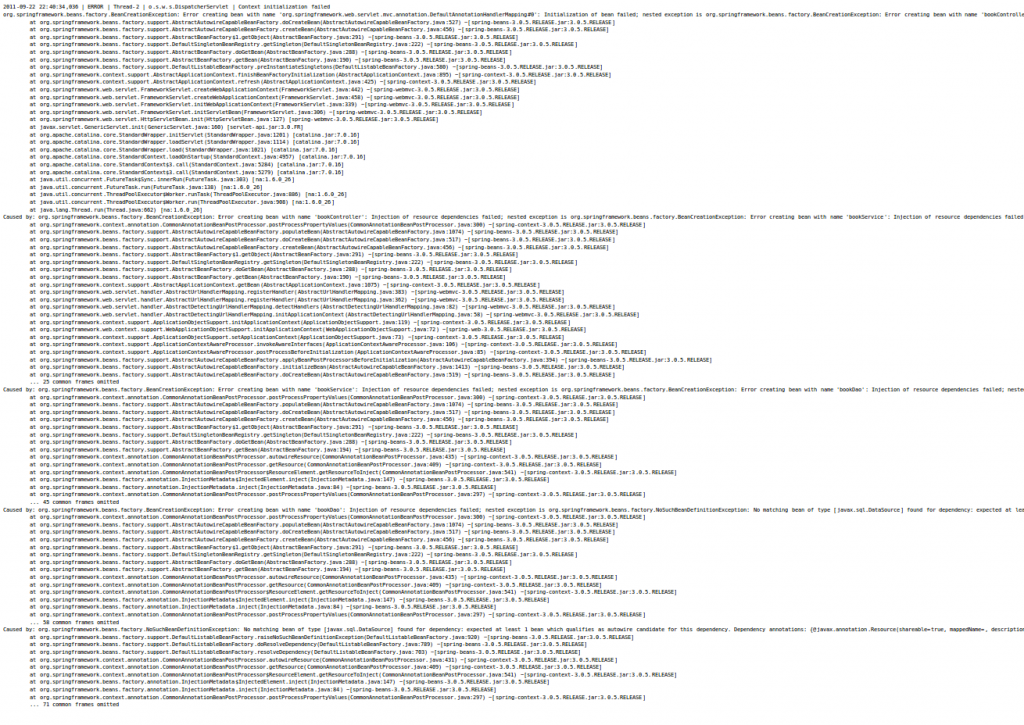
So as a developer you get to spend a few hours a day trawling through log files and deciphering stack traces to find out why Bob in accounting saw two paypal payments instead of one or something. Your task, if you choose to accept it, is to try to stop your eyes glazing over whilst poring over megabytes of irrelevant crap.
My typical stack trace looks a bit like this:
A Contrived Example
org.springframework.jdbc.BadSqlGrammarException: StatementCallback; bad SQL grammar [SELECT flarge FROM tableOfFlonges]; nested exception is com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: Unknown column 'flarge' in 'field list' at org.springframework.jdbc.support.SQLErrorCodeSQLExceptionTranslator.doTranslate(SQLErrorCodeSQLExceptionTranslator.java:220) at org.springframework.jdbc.support.AbstractFallbackSQLExceptionTranslator.translate(AbstractFallbackSQLExceptionTranslator.java:72) at org.springframework.jdbc.core.JdbcTemplate.execute(JdbcTemplate.java:407) at org.springframework.jdbc.core.JdbcTemplate.query(JdbcTemplate.java:458) at org.springframework.jdbc.core.JdbcTemplate.query(JdbcTemplate.java:466) at org.springframework.jdbc.core.JdbcTemplate.queryForList(JdbcTemplate.java:497) at com.contrived.web.action.MainAction.execute(MainAction.java:33) at org.apache.struts.action.RequestProcessor.processActionPerform(RequestProcessor.java:425) at com.randomnoun.common.webapp.struts.CustomRequestProcessor.processActionPerform(CustomRequestProcessor.java:606) at org.apache.struts.action.RequestProcessor.process(RequestProcessor.java:228) at com.randomnoun.common.webapp.struts.CustomRequestProcessor.process(CustomRequestProcessor.java:723) at org.apache.struts.action.ActionServlet.process(ActionServlet.java:1913) at org.apache.struts.action.ActionServlet.doGet(ActionServlet.java:449) at com.randomnoun.common.webapp.struts.CustomActionServlet.doGet(CustomActionServlet.java:55) at javax.servlet.http.HttpServlet.service(HttpServlet.java:621) at javax.servlet.http.HttpServlet.service(HttpServlet.java:722) at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305) at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210) at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:224) at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:169) at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472) at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168) at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:98) at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:927) at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118) at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407) at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:987) at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:579) at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:309) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1110) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:603) at java.lang.Thread.run(Thread.java:722) Caused by: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: Unknown column 'flarge' in 'field list' at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:57) at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45) at java.lang.reflect.Constructor.newInstance(Constructor.java:525) at com.mysql.jdbc.Util.handleNewInstance(Util.java:406) at com.mysql.jdbc.Util.getInstance(Util.java:381) at com.mysql.jdbc.SQLError.createSQLException(SQLError.java:1051) at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3563) at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3495) at com.mysql.jdbc.MysqlIO.sendCommand(MysqlIO.java:1959) at com.mysql.jdbc.MysqlIO.sqlQueryDirect(MysqlIO.java:2113) at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2687) at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2616) at com.mysql.jdbc.StatementImpl.executeQuery(StatementImpl.java:1464) at org.springframework.jdbc.core.JdbcTemplate$1QueryStatementCallback.doInStatement(JdbcTemplate.java:443) at org.springframework.jdbc.core.JdbcTemplate.execute(JdbcTemplate.java:396) ... 29 more
Which you will immediately notice from the first line is because the SQL statement
SELECT flarge FROM tableOfFlonges
is grammatically incorrect.
All you have to do now is find out which bit of code you need to modify in order to fix the bug.
One of the nice, yet underrated things about Java, is that you determine fairly quickly whose code is running, due to the use of packages in the class names. In this example, there’s code from:
- the particular client I’m working for (
com.contrived
), - my own code and libraries (
com.randomnoun
), - the Apache Tomcat application server (
com.apache.tomcat
) - the MySQL JDBC library (
com.mysql
) - a JDBC abstraction mechanism (
com.springframework.jdbc
) - the struts MVC framework (
com.apache.struts
) - etc
What I would like though is something more like the following, in which I assume for the time being that the fault in the code more often than not lies with something that I’ve written.
A Contrived Example with Special Sauce
org.springframework.jdbc.BadSqlGrammarException: StatementCallback; bad SQL grammar [SELECT flarge FROM tableOfFlonges]; nested exception is com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: Unknown column 'flarge' in 'field list' at org.springframework.jdbc.support.SQLErrorCodeSQLExceptionTranslator.doTranslate(SQLErrorCodeSQLExceptionTranslator.java:220) at org.springframework.jdbc.support.AbstractFallbackSQLExceptionTranslator.translate(AbstractFallbackSQLExceptionTranslator.java:72) at org.springframework.jdbc.core.JdbcTemplate.execute(JdbcTemplate.java:407) at org.springframework.jdbc.core.JdbcTemplate.query(JdbcTemplate.java:458) at org.springframework.jdbc.core.JdbcTemplate.query(JdbcTemplate.java:466) at org.springframework.jdbc.core.JdbcTemplate.queryForList(JdbcTemplate.java:497) at com.contrived.web.action.MainAction.execute(MainAction.java:33, ver 1.7) at org.apache.struts.action.RequestProcessor.processActionPerform(RequestProcessor.java:425) at com.randomnoun.common.webapp.struts.CustomRequestProcessor.processActionPerform(CustomRequestProcessor.java:606, ver 1.23) at org.apache.struts.action.RequestProcessor.process(RequestProcessor.java:228) at com.randomnoun.common.webapp.struts.CustomRequestProcessor.process(CustomRequestProcessor.java:723, ver 1.23) at org.apache.struts.action.ActionServlet.process(ActionServlet.java:1913) at org.apache.struts.action.ActionServlet.doGet(ActionServlet.java:449) at com.randomnoun.common.webapp.struts.CustomActionServlet.doGet(CustomActionServlet.java:55, ver 1.3) at javax.servlet.http.HttpServlet.service(HttpServlet.java:621) at javax.servlet.http.HttpServlet.service(HttpServlet.java:722) at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305) at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210) at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:224) at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:169) at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472) at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168) at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:98) at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:927) at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118) at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407) at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:987) at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:579) at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:307) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1110) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:603) at java.lang.Thread.run(Thread.java:722) Caused by: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: Unknown column 'flarge' in 'field list' at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:57) at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45) at java.lang.reflect.Constructor.newInstance(Constructor.java:525) at com.mysql.jdbc.Util.handleNewInstance(Util.java:406) at com.mysql.jdbc.Util.getInstance(Util.java:381) at com.mysql.jdbc.SQLError.createSQLException(SQLError.java:1051) at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3563) at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3495) at com.mysql.jdbc.MysqlIO.sendCommand(MysqlIO.java:1959) at com.mysql.jdbc.MysqlIO.sqlQueryDirect(MysqlIO.java:2113) at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2687) at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2616) at com.mysql.jdbc.StatementImpl.executeQuery(StatementImpl.java:1464) at org.springframework.jdbc.core.JdbcTemplate$1QueryStatementCallback.doInStatement(JdbcTemplate.java:443) at org.springframework.jdbc.core.JdbcTemplate.execute(JdbcTemplate.java:396) ... 29 more
So what you’re getting here is:
- Something that still looks like a stack trace, unlike some other spectacularly undocumented time-saving toolkits.
- Classes within specified packages (and their sub-packages) are highlighted in the stack trace (in the example above, the packages ‘
com.contrived
‘, ‘com.randomnoun
‘, and ‘jsp
‘) - Each class under developer control contains a version identifier:
com.contrived.web.action.MainAction.execute(MainAction.java:33, ver 1.7)
so you can more easily determine exactly what code is running (which is useful for clients which don’t give you access to production machines, yet expect you to be able to fix problems in production).
In the example stacktrace above, I use CVS revision $Id$s, but you could use CI build numbers, maven version numbers, debian package IDs, those meaningless git strings, or whatever you use to track your source code.
My implementation relies on having the following boilerplate code in every class that I have any control over.
1 2
/** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id$";
/** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id$";
which gets expanded out to this when checked into source control:
1 2
/** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id: ExceptionUtils.java,v 1.8 2012-09-10 21:43:01 knoxg Exp $";
/** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id: ExceptionUtils.java,v 1.8 2012-09-10 21:43:01 knoxg Exp $";
If you decide to use a different identification scheme, then update the
getClassRevision()
method as appropriate. (Or refactor the class to use a ClassRevisionAnnotationFactory, if that’s the sort of thing that keeps you entertained).
I bet you’re just gagging for the code at this stage, so here it is:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 | package com.randomnoun.common; /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * BSD Simplified License. (http://www.randomnoun.com/bsd-simplified.html) */ import java.io.*; import java.lang.reflect.*; import java.util.ArrayList; import java.util.List; /** * Exception utilities class. * * <p>This class contains static utility methods for handling and manipulating exceptions; * the only one you're likely to call being * {@link #getStackTraceWithRevisions(Throwable, ClassLoader, int, String)}, * which extracts CVS revision information from classes to produce an annotated (and highlighted) * stack trace. * * <p>When passing in {@link java.lang.ClassLoader}s to these methods, you may want to try one of * <ul> * <li>this.getClass().getClassLoader() * <li>Thread.currentThread().getContextClassLoader() * </ul> * * @blog http://www.randomnoun.com/wp/2012/12/17/marginally-better-stack-traces/ * @version $Id: ExceptionUtils.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ * @author knoxg */ public class ExceptionUtils { public static final String _revision = "$Id: ExceptionUtils.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $"; /** Perform no stack trace element highlighting */ public static final int HIGHLIGHT_NONE = 0; /** Allow stack trace elements to be highlighted, as text */ public static final int HIGHLIGHT_TEXT = 1; /** Allow stack trace elements to be highlighted, as bold HTML */ public static final int HIGHLIGHT_HTML = 2; /** * Private constructor to prevent instantiation of this class */ private ExceptionUtils() { } /** * Converts an exception's stack trace to a string. If the exception passed * to this function is null, returns the empty string. * * @param e exception * * @return string representation of the exception's stack trace */ public static String getStackTrace(Throwable e) { if (e == null) { return ""; } StringWriter writer = new StringWriter(); e.printStackTrace(new PrintWriter(writer)); return writer.toString(); } /** * Converts an exception's stack trace to a string. Each stack trace element * is annotated to include the CVS revision Id, if it contains a public static * String element containing this information. * * <p>Stack trace elements whose classes begin with the specified highlightPrefix * are also marked, to allow easier debugging. Highlights used can be text * (which will insert the string "=>" before relevent stack trace elements), or * HTML (which will render the stack trace element between <b> and </b> tags. * * <p>If HTML highlighting is enabled, then the exception message is also HTML-escaped. * * @param e exception * @param loader ClassLoader used to read stack trace element revision information * @param highlight One of the HIGHLIGHT_* constants in this class * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return string representation of the exception's stack trace */ public static String getStackTraceWithRevisions(Throwable e, ClassLoader loader, int highlight, String highlightPrefix) { if (e == null) { return "(null)"; } StringBuffer sb = new StringBuffer(); // using reflection to remove runtime dependency on beanshell package try { if (e.getClass().getName().equals("bsh.TargetError")) { Method m; m = e.getClass().getMethod("getTarget"); e = (Throwable) m.invoke(e); } } catch (SecurityException e1) { // ignore - just use original exception } catch (NoSuchMethodException e1) { // ignore - just use original exception } catch (IllegalArgumentException e1) { // ignore - just use original exception } catch (IllegalAccessException e1) { // ignore - just use original exception } catch (InvocationTargetException e1) { // ignore - just use original exception } /* if (e instanceof bsh.TargetError) { e = ((bsh.TargetError)e).getTarget(); } */ String s = e.getClass().getName(); String message = e.getLocalizedMessage(); if (highlight==HIGHLIGHT_HTML) { sb.append(escapeHtml((message != null) ? (s + ": " + message) : s )); } else { sb.append((message != null) ? (s + ": " + message) : s ); } sb.append('\n'); // dump the stack trace for the top-level exception StackTraceElement[] trace = null; trace = e.getStackTrace(); for (int i=0; i < trace.length; i++) { sb.append(getStackTraceElementWithRevision(trace[i], loader, highlight, highlightPrefix) + "\n"); } Throwable cause = getCause(e); if (cause != null) { sb.append(getStackTraceWithRevisionsAsCause(cause, trace, loader, highlight, highlightPrefix)); } return sb.toString(); } /** Returns the 'Caused by...' exception text for a chained exception, performing the * same stack trace element reduction as performed by the built-in {@link java.lang.Throwable#printStackTrace()} * class. * * <p>Note that the notion of 'Suppressed' exceptions introduced in Java 7 is not * supported by this implementation. * * @param e the cause of the original exception * @param causedTrace the original exception trace * @param loader ClassLoader used to read stack trace element revision information * @param highlight One of the HIGHLIGHT_* constants in this class * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return the 'caused by' component of a stack trace */ private static String getStackTraceWithRevisionsAsCause(Throwable e, StackTraceElement[] causedTrace, ClassLoader loader, int highlight, String highlightPrefix) { StringBuffer sb = new StringBuffer(); // Compute number of frames in common between this and caused StackTraceElement[] trace = e.getStackTrace(); int m = trace.length-1; int n = causedTrace.length-1; while (m >= 0 && n >=0 && trace[m].equals(causedTrace[n])) { m--; n--; } int framesInCommon = trace.length - 1 - m; String s = e.getClass().getName(); String message = e.getLocalizedMessage(); sb.append("Caused by: "); if (highlight==HIGHLIGHT_HTML) { sb.append(escapeHtml((message != null) ? (s + ": " + message) : s )); } else { sb.append((message != null) ? (s + ": " + message) : s ); } sb.append("\n"); for (int i=0; i <= m; i++) { sb.append(getStackTraceElementWithRevision(trace[i], loader, highlight, highlightPrefix) + "\n"); } if (framesInCommon != 0) sb.append("\t... " + framesInCommon + " more\n"); // Recurse if we have a cause Throwable ourCause = getCause(e); if (ourCause != null) { sb.append(getStackTraceWithRevisionsAsCause(ourCause, trace, loader, highlight, highlightPrefix)); } return sb.toString(); } /** Returns a single stack trace element as a String, with highlighting * * @param ste the StackTraceElement * @param loader ClassLoader used to read stack trace element revision information * @param highlight One of the HIGHLIGHT_* constants in this class * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return the stack trace element as a String, with highlighting */ private static String getStackTraceElementWithRevision(StackTraceElement ste, ClassLoader loader, int highlight, String highlightPrefix) { // s should be something like: // javax.servlet.http.HttpServlet.service(HttpServlet.java:740) String s; if (highlightPrefix==null || highlight==HIGHLIGHT_NONE) { s = " at " + ste.toString(); } else { boolean isHighlighted = (highlight!=HIGHLIGHT_NONE && isHighlighted(ste.getClassName(), highlightPrefix)); if (isHighlighted && highlight==HIGHLIGHT_HTML) { s = " at <b>" + ste.toString() + "</b>"; } else if (isHighlighted && highlight==HIGHLIGHT_TEXT) { s = " => at " + ste.toString(); } else if (!isHighlighted) { s = " at " + ste.toString(); } else { throw new IllegalArgumentException("Unknown highlight " + highlight); } } int endLocation = s.lastIndexOf(")"); if (endLocation!=-1) { try { // remove inner class info String className = ste.getClassName(); String revision = getClassRevision(loader, className); //System.out.println("Class=" + className + ", revision='" + revision + "'"); s = s.substring(0, endLocation) + ", " + revision + s.substring(endLocation); } catch (Exception e2) { } catch (NoClassDefFoundError ncdfe) { } } return s; } /** Returns true if the provided className matches the highlightPrefix pattern supplied, * false otherwise * * @param className The name of a class (i.e. the class contained in a stack trace element) * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return true if the provided className matches the highlightPrefix pattern supplied, * false otherwise */ private static boolean isHighlighted(String className, String highlightPrefix) { if (highlightPrefix.contains(",")) { String[] prefixes = highlightPrefix.split(","); boolean highlighted = false; for (int i=0; i<prefixes.length && !highlighted; i++) { highlighted = className.startsWith(prefixes[i]); } return highlighted; } else { return className.startsWith(highlightPrefix); } } /** Return the number of elements in the current thread's stack trace (i.e. the height * of the call stack). * * @return the height of the call stack */ public static int getStackDepth() { Throwable t = new Throwable(); return t.getStackTrace().length; } /** Returns a string describing the CVS revision number of a class. The class * is initialised as part of this process, which may cause a number of * exceptions to be thrown. * * @param loader The classLoader to use * @param className The class we wish to retrieve * * @return The CVS revision string, in the form "ver 1.234". * * @throws ClassNotFoundException * @throws SecurityException * @throws NoSuchFieldException * @throws IllegalArgumentException * @throws IllegalAccessException */ public static String getClassRevision(ClassLoader loader, String className) throws ClassNotFoundException, SecurityException, NoSuchFieldException, IllegalArgumentException, IllegalAccessException { if (className.indexOf('$')!=-1) { className = className.substring(0, className.indexOf('$')); } Class clazz = Class.forName(className, true, loader); Field field = clazz.getField("_revision"); String revision = (String) field.get(null); // remove rest of $Id: ExceptionUtils.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ text int pos = revision.indexOf(",v "); if (pos != -1) { revision = revision.substring(pos + 3); pos = revision.indexOf(' '); revision = "ver " + revision.substring(0, pos); } return revision; } /** An alternate implementation of {@link #getClassRevision(ClassLoader, String)}, * which searches the raw bytecode of the class, rather than using Java reflection. * May be a bit more robust. * * @TODO implement this * * @param loader Classloader to use * @param className Class to load * * @return The CVS revision string, in the form "ver 1.234". * * @throws ClassNotFoundException * @throws SecurityException * @throws NoSuchFieldException * @throws IllegalArgumentException * @throws IllegalAccessException */ public static String getClassRevision2(ClassLoader loader, String className) throws ClassNotFoundException, SecurityException, NoSuchFieldException, IllegalArgumentException, IllegalAccessException { if (className.indexOf('$')!=-1) { className = className.substring(0, className.indexOf('$')); } String file = className.replace('.', '/') + ".class"; InputStream is = loader.getResourceAsStream(file); // read up to '$Id:' text throw new UnsupportedOperationException("Not implemented"); } /** Returns a list of all the messages contained within a exception (following the causal * chain of the supplied exception). This may be more useful to and end-user, since it * should not contain any references to Java class names. * * @param throwable an exception * * @return a List of Strings */ public static List<String> getStackTraceSummary(Throwable throwable) { List<String> result = new ArrayList<String>(); while (throwable!=null) { result.add(throwable.getMessage()); throwable = getCause(throwable); // I think some RemoteExceptions have non-standard caused-by chains as well... if (throwable==null) { if (throwable instanceof java.sql.SQLException) { throwable = ((java.sql.SQLException) throwable).getNextException(); } } } return result; } /** Returns the cause of an exception, or null if not known. * If the exception is a a <code>bsh.TargetError</code>, then the cause is determined * by calling the <code>getTarget()</code> method, otherwise this method will * return the same value returned by the standard Exception * <code>getCause()</code> method. * * @param e the cause of an exception, or null if not known. * * @return the cause of an exception, or null if not known. */ private static Throwable getCause(Throwable e) { Throwable cause = null; if (e.getClass().getName().equals("bsh.TargetError")) { try { if (e.getClass().getName().equals("bsh.TargetError")) { Method m; m = e.getClass().getMethod("getTarget"); cause = (Throwable) m.invoke(e); } } catch (SecurityException e1) { // ignore - just use original exception } catch (NoSuchMethodException e1) { // ignore - just use original exception } catch (IllegalArgumentException e1) { // ignore - just use original exception } catch (IllegalAccessException e1) { // ignore - just use original exception } catch (InvocationTargetException e1) { // ignore - just use original exception } } else { cause = e.getCause(); } return cause; } /** * Returns the HTML-escaped form of a string. Any <tt>&</tt>, * <tt><</tt>, <tt>></tt>, and <tt>"</tt> characters are converted to * <tt>&amp;</tt>, <tt>&lt;<tt>, <tt>&gt;<tt>, and * <tt>&quot;</tt> respectively. * * @param string the string to convert * * @return the HTML-escaped form of the string */ static public String escapeHtml(String string) { if (string == null) { return ""; } char c; StringBuffer sb = new StringBuffer(string.length()); for (int i = 0; i < string.length(); i++) { c = string.charAt(i); switch (c) { case '&': sb.append("&"); break; case '<': sb.append("<"); break; case '>': sb.append(">"); break; case '\"': sb.append("""); break; default: sb.append(c); } } return sb.toString(); } } |
package com.randomnoun.common; /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * BSD Simplified License. (http://www.randomnoun.com/bsd-simplified.html) */ import java.io.*; import java.lang.reflect.*; import java.util.ArrayList; import java.util.List; /** * Exception utilities class. * * <p>This class contains static utility methods for handling and manipulating exceptions; * the only one you're likely to call being * {@link #getStackTraceWithRevisions(Throwable, ClassLoader, int, String)}, * which extracts CVS revision information from classes to produce an annotated (and highlighted) * stack trace. * * <p>When passing in {@link java.lang.ClassLoader}s to these methods, you may want to try one of * <ul> * <li>this.getClass().getClassLoader() * <li>Thread.currentThread().getContextClassLoader() * </ul> * * @blog http://www.randomnoun.com/wp/2012/12/17/marginally-better-stack-traces/ * @version $Id: ExceptionUtils.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ * @author knoxg */ public class ExceptionUtils { public static final String _revision = "$Id: ExceptionUtils.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $"; /** Perform no stack trace element highlighting */ public static final int HIGHLIGHT_NONE = 0; /** Allow stack trace elements to be highlighted, as text */ public static final int HIGHLIGHT_TEXT = 1; /** Allow stack trace elements to be highlighted, as bold HTML */ public static final int HIGHLIGHT_HTML = 2; /** * Private constructor to prevent instantiation of this class */ private ExceptionUtils() { } /** * Converts an exception's stack trace to a string. If the exception passed * to this function is null, returns the empty string. * * @param e exception * * @return string representation of the exception's stack trace */ public static String getStackTrace(Throwable e) { if (e == null) { return ""; } StringWriter writer = new StringWriter(); e.printStackTrace(new PrintWriter(writer)); return writer.toString(); } /** * Converts an exception's stack trace to a string. Each stack trace element * is annotated to include the CVS revision Id, if it contains a public static * String element containing this information. * * <p>Stack trace elements whose classes begin with the specified highlightPrefix * are also marked, to allow easier debugging. Highlights used can be text * (which will insert the string "=>" before relevent stack trace elements), or * HTML (which will render the stack trace element between <b> and </b> tags. * * <p>If HTML highlighting is enabled, then the exception message is also HTML-escaped. * * @param e exception * @param loader ClassLoader used to read stack trace element revision information * @param highlight One of the HIGHLIGHT_* constants in this class * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return string representation of the exception's stack trace */ public static String getStackTraceWithRevisions(Throwable e, ClassLoader loader, int highlight, String highlightPrefix) { if (e == null) { return "(null)"; } StringBuffer sb = new StringBuffer(); // using reflection to remove runtime dependency on beanshell package try { if (e.getClass().getName().equals("bsh.TargetError")) { Method m; m = e.getClass().getMethod("getTarget"); e = (Throwable) m.invoke(e); } } catch (SecurityException e1) { // ignore - just use original exception } catch (NoSuchMethodException e1) { // ignore - just use original exception } catch (IllegalArgumentException e1) { // ignore - just use original exception } catch (IllegalAccessException e1) { // ignore - just use original exception } catch (InvocationTargetException e1) { // ignore - just use original exception } /* if (e instanceof bsh.TargetError) { e = ((bsh.TargetError)e).getTarget(); } */ String s = e.getClass().getName(); String message = e.getLocalizedMessage(); if (highlight==HIGHLIGHT_HTML) { sb.append(escapeHtml((message != null) ? (s + ": " + message) : s )); } else { sb.append((message != null) ? (s + ": " + message) : s ); } sb.append('\n'); // dump the stack trace for the top-level exception StackTraceElement[] trace = null; trace = e.getStackTrace(); for (int i=0; i < trace.length; i++) { sb.append(getStackTraceElementWithRevision(trace[i], loader, highlight, highlightPrefix) + "\n"); } Throwable cause = getCause(e); if (cause != null) { sb.append(getStackTraceWithRevisionsAsCause(cause, trace, loader, highlight, highlightPrefix)); } return sb.toString(); } /** Returns the 'Caused by...' exception text for a chained exception, performing the * same stack trace element reduction as performed by the built-in {@link java.lang.Throwable#printStackTrace()} * class. * * <p>Note that the notion of 'Suppressed' exceptions introduced in Java 7 is not * supported by this implementation. * * @param e the cause of the original exception * @param causedTrace the original exception trace * @param loader ClassLoader used to read stack trace element revision information * @param highlight One of the HIGHLIGHT_* constants in this class * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return the 'caused by' component of a stack trace */ private static String getStackTraceWithRevisionsAsCause(Throwable e, StackTraceElement[] causedTrace, ClassLoader loader, int highlight, String highlightPrefix) { StringBuffer sb = new StringBuffer(); // Compute number of frames in common between this and caused StackTraceElement[] trace = e.getStackTrace(); int m = trace.length-1; int n = causedTrace.length-1; while (m >= 0 && n >=0 && trace[m].equals(causedTrace[n])) { m--; n--; } int framesInCommon = trace.length - 1 - m; String s = e.getClass().getName(); String message = e.getLocalizedMessage(); sb.append("Caused by: "); if (highlight==HIGHLIGHT_HTML) { sb.append(escapeHtml((message != null) ? (s + ": " + message) : s )); } else { sb.append((message != null) ? (s + ": " + message) : s ); } sb.append("\n"); for (int i=0; i <= m; i++) { sb.append(getStackTraceElementWithRevision(trace[i], loader, highlight, highlightPrefix) + "\n"); } if (framesInCommon != 0) sb.append("\t... " + framesInCommon + " more\n"); // Recurse if we have a cause Throwable ourCause = getCause(e); if (ourCause != null) { sb.append(getStackTraceWithRevisionsAsCause(ourCause, trace, loader, highlight, highlightPrefix)); } return sb.toString(); } /** Returns a single stack trace element as a String, with highlighting * * @param ste the StackTraceElement * @param loader ClassLoader used to read stack trace element revision information * @param highlight One of the HIGHLIGHT_* constants in this class * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return the stack trace element as a String, with highlighting */ private static String getStackTraceElementWithRevision(StackTraceElement ste, ClassLoader loader, int highlight, String highlightPrefix) { // s should be something like: // javax.servlet.http.HttpServlet.service(HttpServlet.java:740) String s; if (highlightPrefix==null || highlight==HIGHLIGHT_NONE) { s = " at " + ste.toString(); } else { boolean isHighlighted = (highlight!=HIGHLIGHT_NONE && isHighlighted(ste.getClassName(), highlightPrefix)); if (isHighlighted && highlight==HIGHLIGHT_HTML) { s = " at <b>" + ste.toString() + "</b>"; } else if (isHighlighted && highlight==HIGHLIGHT_TEXT) { s = " => at " + ste.toString(); } else if (!isHighlighted) { s = " at " + ste.toString(); } else { throw new IllegalArgumentException("Unknown highlight " + highlight); } } int endLocation = s.lastIndexOf(")"); if (endLocation!=-1) { try { // remove inner class info String className = ste.getClassName(); String revision = getClassRevision(loader, className); //System.out.println("Class=" + className + ", revision='" + revision + "'"); s = s.substring(0, endLocation) + ", " + revision + s.substring(endLocation); } catch (Exception e2) { } catch (NoClassDefFoundError ncdfe) { } } return s; } /** Returns true if the provided className matches the highlightPrefix pattern supplied, * false otherwise * * @param className The name of a class (i.e. the class contained in a stack trace element) * @param highlightPrefix A prefix used to determine which stack trace elements are * rendered as being 'important'. (e.g. "<tt>com.randomnoun.common.</tt>"). Multiple * prefixes can be specified, if separated by commas. * * @return true if the provided className matches the highlightPrefix pattern supplied, * false otherwise */ private static boolean isHighlighted(String className, String highlightPrefix) { if (highlightPrefix.contains(",")) { String[] prefixes = highlightPrefix.split(","); boolean highlighted = false; for (int i=0; i<prefixes.length && !highlighted; i++) { highlighted = className.startsWith(prefixes[i]); } return highlighted; } else { return className.startsWith(highlightPrefix); } } /** Return the number of elements in the current thread's stack trace (i.e. the height * of the call stack). * * @return the height of the call stack */ public static int getStackDepth() { Throwable t = new Throwable(); return t.getStackTrace().length; } /** Returns a string describing the CVS revision number of a class. The class * is initialised as part of this process, which may cause a number of * exceptions to be thrown. * * @param loader The classLoader to use * @param className The class we wish to retrieve * * @return The CVS revision string, in the form "ver 1.234". * * @throws ClassNotFoundException * @throws SecurityException * @throws NoSuchFieldException * @throws IllegalArgumentException * @throws IllegalAccessException */ public static String getClassRevision(ClassLoader loader, String className) throws ClassNotFoundException, SecurityException, NoSuchFieldException, IllegalArgumentException, IllegalAccessException { if (className.indexOf('$')!=-1) { className = className.substring(0, className.indexOf('$')); } Class clazz = Class.forName(className, true, loader); Field field = clazz.getField("_revision"); String revision = (String) field.get(null); // remove rest of $Id: ExceptionUtils.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ text int pos = revision.indexOf(",v "); if (pos != -1) { revision = revision.substring(pos + 3); pos = revision.indexOf(' '); revision = "ver " + revision.substring(0, pos); } return revision; } /** An alternate implementation of {@link #getClassRevision(ClassLoader, String)}, * which searches the raw bytecode of the class, rather than using Java reflection. * May be a bit more robust. * * @TODO implement this * * @param loader Classloader to use * @param className Class to load * * @return The CVS revision string, in the form "ver 1.234". * * @throws ClassNotFoundException * @throws SecurityException * @throws NoSuchFieldException * @throws IllegalArgumentException * @throws IllegalAccessException */ public static String getClassRevision2(ClassLoader loader, String className) throws ClassNotFoundException, SecurityException, NoSuchFieldException, IllegalArgumentException, IllegalAccessException { if (className.indexOf('$')!=-1) { className = className.substring(0, className.indexOf('$')); } String file = className.replace('.', '/') + ".class"; InputStream is = loader.getResourceAsStream(file); // read up to '$Id:' text throw new UnsupportedOperationException("Not implemented"); } /** Returns a list of all the messages contained within a exception (following the causal * chain of the supplied exception). This may be more useful to and end-user, since it * should not contain any references to Java class names. * * @param throwable an exception * * @return a List of Strings */ public static List<String> getStackTraceSummary(Throwable throwable) { List<String> result = new ArrayList<String>(); while (throwable!=null) { result.add(throwable.getMessage()); throwable = getCause(throwable); // I think some RemoteExceptions have non-standard caused-by chains as well... if (throwable==null) { if (throwable instanceof java.sql.SQLException) { throwable = ((java.sql.SQLException) throwable).getNextException(); } } } return result; } /** Returns the cause of an exception, or null if not known. * If the exception is a a <code>bsh.TargetError</code>, then the cause is determined * by calling the <code>getTarget()</code> method, otherwise this method will * return the same value returned by the standard Exception * <code>getCause()</code> method. * * @param e the cause of an exception, or null if not known. * * @return the cause of an exception, or null if not known. */ private static Throwable getCause(Throwable e) { Throwable cause = null; if (e.getClass().getName().equals("bsh.TargetError")) { try { if (e.getClass().getName().equals("bsh.TargetError")) { Method m; m = e.getClass().getMethod("getTarget"); cause = (Throwable) m.invoke(e); } } catch (SecurityException e1) { // ignore - just use original exception } catch (NoSuchMethodException e1) { // ignore - just use original exception } catch (IllegalArgumentException e1) { // ignore - just use original exception } catch (IllegalAccessException e1) { // ignore - just use original exception } catch (InvocationTargetException e1) { // ignore - just use original exception } } else { cause = e.getCause(); } return cause; } /** * Returns the HTML-escaped form of a string. Any <tt>&</tt>, * <tt><</tt>, <tt>></tt>, and <tt>"</tt> characters are converted to * <tt>&amp;</tt>, <tt>&lt;<tt>, <tt>&gt;<tt>, and * <tt>&quot;</tt> respectively. * * @param string the string to convert * * @return the HTML-escaped form of the string */ static public String escapeHtml(String string) { if (string == null) { return ""; } char c; StringBuffer sb = new StringBuffer(string.length()); for (int i = 0; i < string.length(); i++) { c = string.charAt(i); switch (c) { case '&': sb.append("&"); break; case '<': sb.append("<"); break; case '>': sb.append(">"); break; case '\"': sb.append("""); break; default: sb.append(c); } } return sb.toString(); } }
and some unit test classes that you’ll never look at:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | package com.randomnoun.common; /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * BSD Simplified License. (http://www.randomnoun.com/bsd-simplified.html) */ /** Throwaway class used in ExceptionUtilsTest. * * @see java.lang.Throwable#getStackTrace() * * @author knoxg * @blog http://www.randomnoun.com/wp/2012/12/17/marginally-better-stack-traces/ * @version $Id: Junk.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ * */ public class Junk { /** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id: Junk.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $"; static void a() throws HighLevelException { try { b(); } catch(MidLevelException e) { throw new HighLevelException(e); } } static void b() throws MidLevelException { c(); } static void c() throws MidLevelException { try { d(); } catch(LowLevelException e) { throw new MidLevelException(e); } } static void d() throws LowLevelException { e(); } static void e() throws LowLevelException { throw new LowLevelException(); } public static class InnerClassB1 { // we normally wouldn't revision inner classes // public static String _revision = "Test revision B1"; public static void f() throws LowLevelException { InnerClassB2.g(); } public static class InnerClassB2 { // we normally wouldn't revision inner classes // public static String _revision = "Test revision B2"; public static void g() throws LowLevelException { throw new LowLevelException(); } } } } /** Utility exception class */ class HighLevelException extends Exception { HighLevelException(Throwable cause) { super(cause); } } /** Utility exception class */ class MidLevelException extends Exception { MidLevelException(Throwable cause) { super("<middle>", cause); } } /** Utility exception class */ class LowLevelException extends Exception { } |
package com.randomnoun.common; /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * BSD Simplified License. (http://www.randomnoun.com/bsd-simplified.html) */ /** Throwaway class used in ExceptionUtilsTest. * * @see java.lang.Throwable#getStackTrace() * * @author knoxg * @blog http://www.randomnoun.com/wp/2012/12/17/marginally-better-stack-traces/ * @version $Id: Junk.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ * */ public class Junk { /** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id: Junk.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $"; static void a() throws HighLevelException { try { b(); } catch(MidLevelException e) { throw new HighLevelException(e); } } static void b() throws MidLevelException { c(); } static void c() throws MidLevelException { try { d(); } catch(LowLevelException e) { throw new MidLevelException(e); } } static void d() throws LowLevelException { e(); } static void e() throws LowLevelException { throw new LowLevelException(); } public static class InnerClassB1 { // we normally wouldn't revision inner classes // public static String _revision = "Test revision B1"; public static void f() throws LowLevelException { InnerClassB2.g(); } public static class InnerClassB2 { // we normally wouldn't revision inner classes // public static String _revision = "Test revision B2"; public static void g() throws LowLevelException { throw new LowLevelException(); } } } } /** Utility exception class */ class HighLevelException extends Exception { HighLevelException(Throwable cause) { super(cause); } } /** Utility exception class */ class MidLevelException extends Exception { MidLevelException(Throwable cause) { super("<middle>", cause); } } /** Utility exception class */ class LowLevelException extends Exception { }
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 | package com.randomnoun.common; /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * BSD Simplified License. (http://www.randomnoun.com/bsd-simplified.html) */ import java.io.ByteArrayOutputStream; import java.io.PrintStream; import java.util.ArrayList; import java.util.List; import java.util.regex.Pattern; import junit.framework.TestCase; /** Test class for Exception Utils * * @author knoxg * @blog http://www.randomnoun.com/wp/2012/12/17/marginally-better-stack-traces/ * @version $Id: ExceptionUtilsTest.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ */ public class ExceptionUtilsTest extends TestCase { /** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id: ExceptionUtilsTest.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $"; public void testGetStackTrace() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); // check that our getStackTrace() method matches java's printStackTrace() method assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); } public void testGetStackTraceWithRevisionsNoHighlight() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); // check that our getStackTrace() method matches java's printStackTrace() method /* This is what we expect when run in the Eclipse UI We need to generalise this a bit to take into consideration different test runners, changes in line numbers and changes in version numbers. String expected = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.a(Junk.java:24, ver 1.12)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:55, ver 1.12)\n" + " at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)\n" + " at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57)\n" + " at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)\n" + " at java.lang.reflect.Method.invoke(Method.java:601)\n" + " at junit.framework.TestCase.runTest(TestCase.java:154)\n" + " at junit.framework.TestCase.runBare(TestCase.java:127)\n" + " at junit.framework.TestResult$1.protect(TestResult.java:106)\n" + " at junit.framework.TestResult.runProtected(TestResult.java:124)\n" + " at junit.framework.TestResult.run(TestResult.java:109)\n" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + " at junit.framework.TestSuite.runTest(TestSuite.java:208)\n" + " at junit.framework.TestSuite.run(TestSuite.java:203)\n" + " at org.eclipse.jdt.internal.junit.runner.junit3.JUnit3TestReference.run(JUnit3TestReference.java:130)\n" + " at org.eclipse.jdt.internal.junit.runner.TestExecution.run(TestExecution.java:38)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:467)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:683)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.run(RemoteTestRunner.java:390)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.main(RemoteTestRunner.java:197)\n" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.c(Junk.java:36, ver 1.12)\n" + " at com.randomnoun.common.Junk.b(Junk.java:29, ver 1.12)\n" + " at com.randomnoun.common.Junk.a(Junk.java:22, ver 1.12)\n" + " ... 19 more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:45, ver 1.12)\n" + " at com.randomnoun.common.Junk.d(Junk.java:41, ver 1.12)\n" + " at com.randomnoun.common.Junk.c(Junk.java:34, ver 1.12)\n" + " ... 21 more"; */ expectedRegex = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:[0-9]+)\n" + ".*" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" ; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); expectedRegex = "com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackTraceWithRevisionsText() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " => at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:[0-9]+)\n" + ".*" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" ; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.MidLevelException: <middle>\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*" + "Caused by: com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackTraceWithRevisionsHtml() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " at <b>com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:[0-9]+)\n" + ".*" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + "\t... [0-9]+ more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + "\t... [0-9]+ more\n" ; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.MidLevelException: <middle>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + "\t... [0-9]+ more\n"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackTraceWithRevisionsTextInnerClass() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.InnerClassB1.f(); } catch(LowLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk\\$InnerClassB1\\$InnerClassB2.g(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk\\$InnerClassB1.f(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsTextInnerClass(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.InnerClassB1.InnerClassB2.g(); } catch(LowLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk\\$InnerClassB1\\$InnerClassB2.g(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsTextInnerClass(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackDepth() { // not the sort of thing you can test, really } public void testGetStackTraceSummary() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; List<String> summary = null; List<String> expectedSummary = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add("com.randomnoun.common.MidLevelException: <middle>"); expectedSummary.add("<middle>"); expectedSummary.add(null); assertEquals(expectedSummary, summary); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add("<middle>"); expectedSummary.add(null); assertEquals(expectedSummary, summary); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add(null); assertEquals(expectedSummary, summary); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add(null); assertEquals(expectedSummary, summary); } } |
package com.randomnoun.common; /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * BSD Simplified License. (http://www.randomnoun.com/bsd-simplified.html) */ import java.io.ByteArrayOutputStream; import java.io.PrintStream; import java.util.ArrayList; import java.util.List; import java.util.regex.Pattern; import junit.framework.TestCase; /** Test class for Exception Utils * * @author knoxg * @blog http://www.randomnoun.com/wp/2012/12/17/marginally-better-stack-traces/ * @version $Id: ExceptionUtilsTest.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $ */ public class ExceptionUtilsTest extends TestCase { /** A revision marker to be used in exception stack traces. */ public static final String _revision = "$Id: ExceptionUtilsTest.java,v 1.2 2013-09-24 02:37:09 knoxg Exp $"; public void testGetStackTrace() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); // check that our getStackTrace() method matches java's printStackTrace() method assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); } public void testGetStackTraceWithRevisionsNoHighlight() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); // check that our getStackTrace() method matches java's printStackTrace() method /* This is what we expect when run in the Eclipse UI We need to generalise this a bit to take into consideration different test runners, changes in line numbers and changes in version numbers. String expected = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.a(Junk.java:24, ver 1.12)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:55, ver 1.12)\n" + " at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)\n" + " at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57)\n" + " at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)\n" + " at java.lang.reflect.Method.invoke(Method.java:601)\n" + " at junit.framework.TestCase.runTest(TestCase.java:154)\n" + " at junit.framework.TestCase.runBare(TestCase.java:127)\n" + " at junit.framework.TestResult$1.protect(TestResult.java:106)\n" + " at junit.framework.TestResult.runProtected(TestResult.java:124)\n" + " at junit.framework.TestResult.run(TestResult.java:109)\n" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + " at junit.framework.TestSuite.runTest(TestSuite.java:208)\n" + " at junit.framework.TestSuite.run(TestSuite.java:203)\n" + " at org.eclipse.jdt.internal.junit.runner.junit3.JUnit3TestReference.run(JUnit3TestReference.java:130)\n" + " at org.eclipse.jdt.internal.junit.runner.TestExecution.run(TestExecution.java:38)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:467)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:683)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.run(RemoteTestRunner.java:390)\n" + " at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.main(RemoteTestRunner.java:197)\n" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.c(Junk.java:36, ver 1.12)\n" + " at com.randomnoun.common.Junk.b(Junk.java:29, ver 1.12)\n" + " at com.randomnoun.common.Junk.a(Junk.java:22, ver 1.12)\n" + " ... 19 more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:45, ver 1.12)\n" + " at com.randomnoun.common.Junk.d(Junk.java:41, ver 1.12)\n" + " at com.randomnoun.common.Junk.c(Junk.java:34, ver 1.12)\n" + " ... 21 more"; */ expectedRegex = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:[0-9]+)\n" + ".*" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" ; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); expectedRegex = "com.randomnoun.common.MidLevelException: <middle>\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_NONE, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsNoHighlight(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackTraceWithRevisionsText() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " => at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:[0-9]+)\n" + ".*" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n" ; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.MidLevelException: <middle>\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*" + "Caused by: com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)\n" + "\t... [0-9]+ more\n"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsText(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackTraceWithRevisionsHtml() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.HighLevelException: com.randomnoun.common.MidLevelException: <middle>\n" + " at <b>com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:[0-9]+)\n" + ".*" + "Caused by: com.randomnoun.common.MidLevelException: <middle>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.a(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + "\t... [0-9]+ more\n" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + "\t... [0-9]+ more\n" ; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.MidLevelException: <middle>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.b(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*" + "Caused by: com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.c(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + "\t... [0-9]+ more\n"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.Junk.d(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " at <b>com.randomnoun.common.Junk.e(Junk.java:[0-9]+, ver [0-9.]+)</b>\n" + " at <b>com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsHtml(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)</b>\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackTraceWithRevisionsTextInnerClass() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; String highlightedStackTrace = null; String expectedRegex = null; Pattern expectedPattern = null; try { Junk.InnerClassB1.f(); } catch(LowLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); assertEquals(baos.toString(), ExceptionUtils.getStackTrace(e)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk\\$InnerClassB1\\$InnerClassB2.g(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.Junk\\$InnerClassB1.f(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsTextInnerClass(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); try { Junk.InnerClassB1.InnerClassB2.g(); } catch(LowLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); highlightedStackTrace = ExceptionUtils.getStackTraceWithRevisions(e, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_TEXT, "com.randomnoun."); expectedRegex = "com.randomnoun.common.LowLevelException\n" + " => at com.randomnoun.common.Junk\\$InnerClassB1\\$InnerClassB2.g(Junk.java:[0-9]+, ver [0-9.]+)\n" + " => at com.randomnoun.common.ExceptionUtilsTest.testGetStackTraceWithRevisionsTextInnerClass(ExceptionUtilsTest.java:[0-9]+, ver [0-9.]+)\n" + ".*" + " at junit.framework.TestCase.run(TestCase.java:118)\n" + ".*"; expectedRegex = expectedRegex.replaceAll("\\(", "\\\\("); // regex-escape grouping operators expectedRegex = expectedRegex.replaceAll("\\)", "\\\\)"); expectedPattern = Pattern.compile(expectedRegex, Pattern.DOTALL); assertTrue("Incorrect stacktrace:\n" + highlightedStackTrace, expectedPattern.matcher(highlightedStackTrace).find()); } public void testGetStackDepth() { // not the sort of thing you can test, really } public void testGetStackTraceSummary() { ByteArrayOutputStream baos = new ByteArrayOutputStream(); Exception e = null; List<String> summary = null; List<String> expectedSummary = null; try { Junk.a(); } catch(HighLevelException hle) { e = hle; } e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add("com.randomnoun.common.MidLevelException: <middle>"); expectedSummary.add("<middle>"); expectedSummary.add(null); assertEquals(expectedSummary, summary); try { Junk.b(); } catch(MidLevelException mle) { e = mle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add("<middle>"); expectedSummary.add(null); assertEquals(expectedSummary, summary); try { Junk.d(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add(null); assertEquals(expectedSummary, summary); try { Junk.e(); } catch(LowLevelException lle) { e = lle; } baos.reset(); e.printStackTrace(new PrintStream(baos)); summary = ExceptionUtils.getStackTraceSummary(e); expectedSummary = new ArrayList<String>(); expectedSummary.add(null); assertEquals(expectedSummary, summary); } }
And a JSP errorPage handler which uses it:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | <?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd"> <%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" import="java.util.*,java.text.*,com.randomnoun.common.ExceptionUtils" %> <%-- /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * <a rel="license" href="http://creativecommons.org/licenses/by/3.0/">Creative Commons Attribution 3.0 Unported License</a>. */ --%> <% response.setStatus(500); %> <html xmlns="http://www.w3.org/1999/xhtml"> <!-- $Id: errorPage.jsp,v 1.2 2013-01-08 19:28:23 knoxg Exp $ --> <head> <title>Error</title> </head> <body style="font-family: Arial;"> <% Throwable exception = (Throwable) request.getAttribute("javax.servlet.error.exception"); String message = (String) request.getAttribute("javax.servlet.error.message"); if (exception==null) { exception = (Throwable) request.getAttribute("javax.servlet.jsp.jspException"); if (exception!=null) { message = exception.getMessage(); } } SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss zzzz"); String timeString = sdf.format(new Date()); /* if (exception instanceof RemoteException) { exception = ((RemoteException) exception).detail; } */ %> <table style="border: red solid 1px; background-color: #FFFEBB; margin:10px; font-size: 8pt"> <tr> <td valign="top"><img align="left" src="image/information.gif" /></td> <td valign="top"> <b>An error has occurred in the application</b> <br/><br/> <b>Message:</b> <%= message %> <br/><br/> <b>Request URI:</b> <%= request.getAttribute("javax.servlet.error.request_uri") %> <br/><b>Request Time:</b> <%= timeString %> <%-- should only display this in development mode, otherwise log or email it --%> <br/><br/> <% if (exception!=null) { %> <b>Stack trace:</b> <a href="javascript:void();" onclick="x = document.getElementById('stackTrace').style; x.display = (x.display=='block' ? 'none' : 'block');">Click to display</a> <div id="stackTrace" style="display:none"><pre><%= ExceptionUtils.getStackTraceWithRevisions(exception, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun.,com.example.contrived.,org.apache.jsp.") %></pre></div> <% } %> </td> </tr> </table> </body> </html> <% out.flush(); /* if (request.getAttribute("sentAlarm")==null) { ExceptionHandler.sendApplicationAlarm(request, exception, "JSP"); } */ %> |
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd"> <%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" import="java.util.*,java.text.*,com.randomnoun.common.ExceptionUtils" %> <%-- /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * <a rel="license" href="http://creativecommons.org/licenses/by/3.0/">Creative Commons Attribution 3.0 Unported License</a>. */ --%> <% response.setStatus(500); %> <html xmlns="http://www.w3.org/1999/xhtml"> <!-- $Id: errorPage.jsp,v 1.2 2013-01-08 19:28:23 knoxg Exp $ --> <head> <title>Error</title> </head> <body style="font-family: Arial;"> <% Throwable exception = (Throwable) request.getAttribute("javax.servlet.error.exception"); String message = (String) request.getAttribute("javax.servlet.error.message"); if (exception==null) { exception = (Throwable) request.getAttribute("javax.servlet.jsp.jspException"); if (exception!=null) { message = exception.getMessage(); } } SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss zzzz"); String timeString = sdf.format(new Date()); /* if (exception instanceof RemoteException) { exception = ((RemoteException) exception).detail; } */ %> <table style="border: red solid 1px; background-color: #FFFEBB; margin:10px; font-size: 8pt"> <tr> <td valign="top"><img align="left" src="image/information.gif" /></td> <td valign="top"> <b>An error has occurred in the application</b> <br/><br/> <b>Message:</b> <%= message %> <br/><br/> <b>Request URI:</b> <%= request.getAttribute("javax.servlet.error.request_uri") %> <br/><b>Request Time:</b> <%= timeString %> <%-- should only display this in development mode, otherwise log or email it --%> <br/><br/> <% if (exception!=null) { %> <b>Stack trace:</b> <a href="javascript:void();" onclick="x = document.getElementById('stackTrace').style; x.display = (x.display=='block' ? 'none' : 'block');">Click to display</a> <div id="stackTrace" style="display:none"><pre><%= ExceptionUtils.getStackTraceWithRevisions(exception, this.getClass().getClassLoader(), ExceptionUtils.HIGHLIGHT_HTML, "com.randomnoun.,com.example.contrived.,org.apache.jsp.") %></pre></div> <% } %> </td> </tr> </table> </body> </html> <% out.flush(); /* if (request.getAttribute("sentAlarm")==null) { ExceptionHandler.sendApplicationAlarm(request, exception, "JSP"); } */ %>
And an example broken JSP:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | <%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" errorPage="misc/errorPage.jsp" %> <%-- /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * <a rel="license" href="http://creativecommons.org/licenses/by/3.0/">Creative Commons Attribution 3.0 Unported License</a>. */ --%> <html> <head> <title>A contrived web application</title> </head> <body> <h2>Hello from contrived-web !</h2> <p>And now for your ClassCastException. <% Comparable thee = "Juliet"; Comparable aSummersDay = new Long(38); // degrees celsius %> thee are <%= thee.compareTo(aSummersDay) > 0 ? "greater" : "less than or equal to" %> a Summer's Day </body> </html> |
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1" errorPage="misc/errorPage.jsp" %> <%-- /* (c) 2013 randomnoun. All Rights Reserved. This work is licensed under a * <a rel="license" href="http://creativecommons.org/licenses/by/3.0/">Creative Commons Attribution 3.0 Unported License</a>. */ --%> <html> <head> <title>A contrived web application</title> </head> <body> <h2>Hello from contrived-web !</h2> <p>And now for your ClassCastException. <% Comparable thee = "Juliet"; Comparable aSummersDay = new Long(38); // degrees celsius %> thee are <%= thee.compareTo(aSummersDay) > 0 ? "greater" : "less than or equal to" %> a Summer's Day </body> </html>
which looks like this when you try to view it in your application server of choice (click the image below to see the actual page).
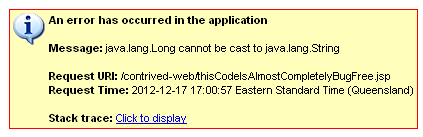
What you could also do if you were really keen would be to generate hrefs in the generated HTML stacktrace to (your favourite web-based source control system), but that’s probably not that useful since
- on a production system you probably shouldn’t be showing stack traces since this may lead your end-users to believe that the website/application isn’t being run by a glistening ball of energy in The Cloud somewhere
- the end-user won’t have permissions to your source control system
- on a development system you probably haven’t checked in the code you’re currently debugging anyway
So there you go. If you find the above code useful then more power to you.
Update 2013-09-25: This code is in the com.randomnoun.common:common-public maven artifact:

Update 2021-01-29: and github:

Related Posts
-
Intruder alert
5 Comments | Jan 28, 2013 -
Bitrot
No Comments | Sep 15, 2013 -
It’s all made out of pipes
3 Comments | Oct 8, 2013 -
Logging
2 Comments | Jan 13, 2013